When creating web applications, we would know one thing that we should always create this app with authentication and security and yes, that's packaged with a login page, this is a redundant code right? and we always integrate login pages with applications and worse, it can also be huge pain in our dev life as we will worry about the security factors such as encryption and much worse, we will need to add more features such as multi factor authentication, Single Sign-On (SSO) , Role Access, Anomaly detection and so on to make our app ironclad against threats.
Thinking of integrating these features is just mind blown …
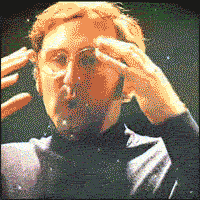
With these instances, why not just create a universal login that a server can provide for our products and all security handling are centralized by a main authentication server, this is what Auth0 comes into part, It simply adds authentication to our applications on the fly by providing a universal login page that will handle all security features. yes that's right! you don't have to worry all the security stuffs and Auth0 will be the one to take car of all that stuff. It also provides the following features that will help our application more secure.
Other Features
• Universal Login for your multiple apps (OIDC Concept)
• Single Sign-on (SSO) capability
• Anomaly Detection
• Multi-Factor Authentication (MFA)
and More....
How does Auth0 really works
Auth0 applies the concepts of OpenID Connect (OIDC) which provides an identity layer that will be executed by an authorization server, the login page acts as the identity layer that will ask users for its credentials, if all provided credentials are valid it will return a valid JSON Web token JWT) in our application, for more understanding let's see the detailed flow for Auth0 as User tries to login.

On the flow chart above, let's say that we have a sample angular application that uses Auth0, the first load of the app will not possess a token and this will call the Auth server to redirect the user on the universal login, after successful login, this will redirect to the angular app together with the user info and valid token and that's basically it now Iet's set this up on our Angular app.
Setting up Auth0
Our first step is to create our account on the following link https://auth0.com/signup, we can use our Github, google or Microsoft account for creation of our account. Auth0 will ask our tenant domain, this will be the name of our server and you can add one or more tenants for the need of your environment.
And that's it we have created our Auth0 account. we should now be able to see our dashboard after all the previous steps, the next step is we need to create an application, click the applications option on the left menu and click create application,

This will let you input you app name select an application type, in this scenario we will select Single Page Web Applications.

And after some loading initiation, we have successfully created our own authentication server! we should now see our application details and provided guides on how to integrate auth0 on different JavaScript frameworks.

Now we have our own authentication server, we can now integrate Auth0 in angular, we would want our Angular app to redirect in our server if no valid token exists. go the settings tab of our Auth0 Server, save the domain and clientid as we will use this in our Angular app.
On our angular application, we will install the Auth0 dependency using the npm command:
npm install @auth0/auth0-angular
after successful installation, we will now import the AuthModule in app.module.ts with the settings of our Auth server.
import { AuthModule } from '@auth0/auth0-angular';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
// Import the module into the application, with configuration
AuthModule.forRoot({
domain: 'dev-9wq4ue47.us.auth0.com',
clientId: 'pXzZ8J4lv05H4jYe3dfqQvR0Z66o7OgK',
redirectUri: 'http://localhost:4200',
scope: 'openid'
}),
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
The domain and clientid are the information to identify what is our authentication server, this is the information we have retrieved under the settings tab, the redirectUri instructs the Authentication server whete redirect after successful login, in this case we want to redirect on http://localhost:4200 on our dev environment.
The next step here is to check if the user is authenticated or not, we will use the AuthService to access the isAuthenticated$ Observable to check if there is an existing token.
constructor(public auth: AuthService) {}
ngOnInit() {
this.auth.isAuthenticated$.subscribe(data => {
if(!data) {
this.auth.loginWithRedirect();
}
});
}
if no valid token is existing, the observable will return false, we want to redirect this on our authentication server to access our login page by calling the function this.auth.loginWithRedirect().

Hooray!! we have successfully configured our Auth0 universal login, sending a valid credential will redirects us back to our Angular app and with that, it is now working smoothly.
Implementing Retrieving User info and Logout
Getting the user info is very simple as the AuthService also provided us the function to do this, we will just call the AuthService.user$ observable and this will return all the user information.
for the logout implementation, we will create a button that calls the logout function provided by AuthService with a JSON parameter containing a returnTo property.
<button (click)="auth.logout({ returnTo: document.location.origin })">
Log out
</button>
an that's it we have successfully implemented Auth0 in Angular, I will not tackle other services provided by Auth0 here in this content but if you want to learn more you can refer to the documentation of Auth0 with the following link: https://auth0.com/docs or you can also join our community here on this link: https://community.auth0.com for more tips and tricks from other experts and my fellow Auth0 Ambassadors.
Thank you for reading my turorial, you can follow my Github: https://github.com/SeijiV13 for more example codes